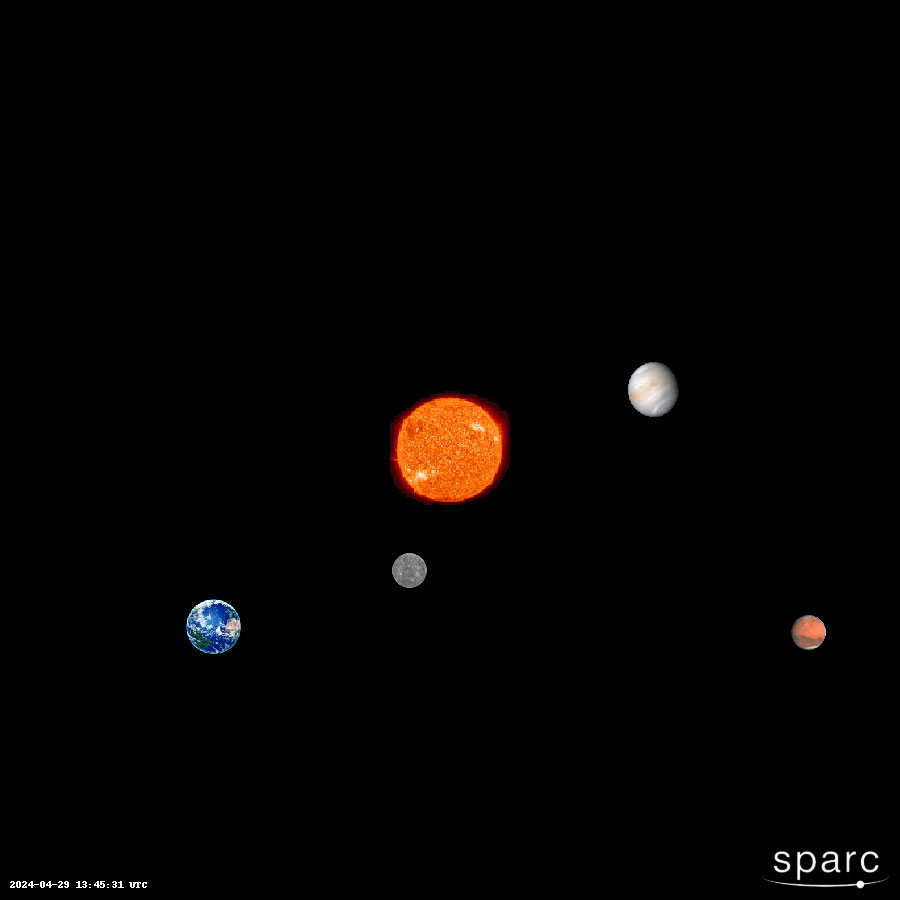
Last summer (2020) the telescope finally got plenty of action due to the excitement over Jupiter and Saturn being easily visible. Previously, I had tried around 10 years ago to view Mars when the telescope was first purchased but it was a disaster. A story for another time.
I got an itch to calculate planet positions and managed to scratch it in a most satisfying way. I dump the main code below as is (sorry, without the resources, comments, etc.) but is relatively straight forward.
from skyfield.api import load
from PIL import Image, ImageDraw
####################################################################
t = load.timescale( ).now( )
####################################################################
planets = load( "de421.bsp" )
planet_positions = [
planets[ "mercury" ].at( t ).position.au ,
planets[ "venus" ].at( t ).position.au ,
planets[ "earth" ].at( t ).position.au ,
planets[ "mars" ].at( t ).position.au ,
]
####################################################################
image_width = 900
image_height = 900
image = Image.new( "RGB" , ( image_width , image_height ) )
draw = ImageDraw.Draw( image )
image_cx = round( image_width / 2 )
image_cy = round( image_height / 2 )
####################################################################
image_sun = Image.open( "sun.png" ).resize( ( 120 , 120 ) , 0 )
image.paste( image_sun , ( image_cx , image_cy ) )
####################################################################
planet_images = [
Image.open( "mercury.png" ).resize( ( 35 , 35 ) , 0 ) ,
Image.open( "venus.png" ).resize( ( 55 , 55 ) , 0 ) ,
Image.open( "earth.png" ).resize( ( 55 , 55 ) , 0 ) ,
Image.open( "mars.png" ).resize( ( 35 , 35 ) , 0 ) ,
]
####################################################################
for i , planet_position in enumerate( planet_positions ) :
px = round( image_cx + ( planet_position[ 0 ] * 300 ) )
py = round( image_cy + ( planet_position[ 1 ] * 300 * -1 ) )
image.paste( planet_images[ i ] , ( px,py ) )
####################################################################
msg = t.utc_strftime( )
msg_w , msg_h = draw.textsize( msg )
draw.text( ( ( image_width - msg_w ) / 2 , image_height - 50 ) , msg , fill = "white" )
####################################################################
image.save( "output.jpg" , quality = "web_high" )
Anyway, this is the first “Labs” post and I’ll keep it short. There should be more posts as I get more fancy ideas. I may publish the full project to GitHub in the near future. Any feedback most welcome especially if I’ve screwed up with the code – I’m no rocket scientist.
I thank Brandon Rhodes for the most elegant Python astronomy library Skyfield. Check it out here: